Ruby on Rails is a web application framework that promotes rapid development. Clients’ demands are ever increasing yet they still expect the same quality of output.
Frameworks, like Rails, help to achieve this; why?… here are some of the reasons:
- The second part of this tutorial: A Simple Twitter App with Ruby on Rails – User Authentication
- The third part of this tutorial: A Simple Twitter App with Ruby on Rails – Building Friendships
- Convention over Configuration (CoC):
This is used to reduce the amount of up-front configuartion. The idea is; if you abide by certain coding conventions, you will have little, to none, configuration to do. - Object-Relational Mapping (ORM):
ORM reducing coupling to the database. This abstraction allows you changed the DBMS provider with little trouble. - Structured Code:
The MVC pattern forces you to organise your code in a clean, structured way. This results in more maintainable code. - Plugins:
Plugins save you from re-inventing the wheel every time you want to add functionality to your app. For instance, making you web app capable of performing searches can be easily added with the acts_as_ferret plugin. There are many more plugins!
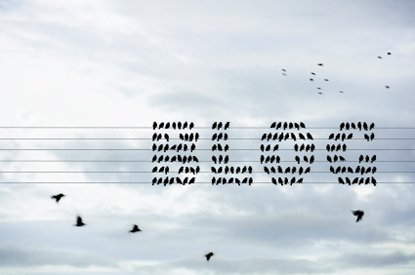
[Editor's note: A must-have for professional Web designers and developers: The Printed Smashing Books Bundle is full of practical insight for your daily work. Get the bundle right away!]
Who is this Tutorial for?
This tutorial is for people who have learnt the basics of Rails and want to take things to the next level. This tutorial is not a beginners guide for getting started with Rails. If you are just starting out with Rails I suggest this article from Six Revisions.
What this Tutorial Covers
In the first part of this three part series, we cover setting up a simple message model, which will hold the messages posted. Further to this, we will learn how to post a message asynchronously, using AJAX.
Basic Application Design

Ok, so you’ve decided to create a “twitter” style micro-blog using Ruby on Rails. First, we need to think about our basic requirements and from this we can model our application.
There are many ways that this can be done, but we will use a simple technique in which you jot down a few paragraphs about how and what the application is expected to do then highlight the nouns. So, lets try it.
My web app should work in a similar way to twitter. Users should be able to register with the site and create short posts. Users should be able to follow other users. Each user should be able to see their own posts plus the users they are following.
Note that I’ve been selective in what nouns I’ve highlighted. You only really need to take notice of the nouns which you feel will need to store data to the database.
I know there is more to twitter than this, but lets leave it simple. As you can see the “nouns”, which will need to store data to the database are “posts” and “users”. So we require two models:
In the first part of the tutorial, we are going to deal with posts only.
- Post
- User
Creating the Project Files
Before we do anything we need to create a project for our twitter web app.
- > rails twitter -d mysql
As you can see, I will be using MySQL as the DBMS, however, feel free to use whatever database you want.
Open the database.yml file in the config folder and modify the password as required. An example is shown below.
- development:
- adapter: mysql
- encoding: utf8
- database: twittest_development
- pool: 5
- username: root
- password: yourpassword
- host: localhost
Now, create the database with the “rake” command.
- > rake db:create
Implementing the basic Message Model

So let’s go right ahead and generate the “Post” model and migrate it.
- > ruby script/generate model post message:text
- > rake db:migrate
Controller
Now, let’s create a controller for the post model.
- > ruby script/generate controller posts
We need to set up some methods for interacting with the model. Edit your “posts_controller.rb” file and add the following methods:
- class PostsController < ApplicationController
- def index
- @posts = Post.all(:order => "created_at DESC")
- respond_to do |format|
- format.html
- end
- end
- def create
- @post = Post.create(:message => params[:message])
- respond_to do |format|
- if @post.save
- format.html { redirect_to posts_path }
- else
- flash[:notice] = "Message failed to save."
- format.html { redirect_to posts_path }
- end
- end
- end
- end
We only need two methods, “index” and “create”. The index method creates an instance variable containing all the posts in descending order. The create method is used to create a new post.
Views
Let’s create the “index” view. First, we’ll create a partial for posts. Create a file called “_post.html.erb” in the views/posts folder and add the code below.
- Posted <%= time_ago_in_words(post.created_at) %> ago
- <%= post.message %>
The index view is now very simple. Create a file called “index.html.erb” in the views/posts folder and add the code below.
- <%= render :partial => @posts %>
Create some Posts
Open a console session and create a few new messages, as shown below.
- > ruby script/console
- Loading development environment (Rails 2.3.2)
- >> Post.create!(:message => "My first post" )
- >> Post.create!(:message => "Post number two!" )
Create a Form for Posts
Obviously you’re not going to get the user to use the console to create messages. So, our next task is to inject some functionality into our web app to allow the user to create messages. Twitter has an input box above the indexed messages, which is used for submitting a new message; We will keep our web app the same.
First, we will create a partial for the form, then we will render that partial at the top of the index view. Create a file called “_message_form.html.erb” in the posts view folder and add the following code:
- <% form_tag(:controller => "posts", :action => "create") do %>
- <%= label_tag(:message, "What are you doing?") %>
- <%= text_area_tag(:message, nil, :size => "44x6") %>
- <%= submit_tag("Update") %>
- <% end %>
Now, we need to modify the index view to render this partial at the top. Open the index.html.erb file and modify the code as follows:
- < %= render :partial => "message_form" %>
- < %= render :partial => @posts %>
For this to work we need to make one last modification. Open the route.rb file and map a new “posts” resource, as shown below. (Note: the comments from this file have been removed).
- ActionController::Routing::Routes.draw do |map|
- map.resources :posts
- map.connect ':controller/:action/:id'
- map.connect ':controller/:action/:id.:format'
- end
This creates a few named routes. If you look back to the “create” method in the posts controller, you’ll see that we make use of the posts_path named route; Defining the posts resource makes this named route available.
So, lets fire up the web server and a see how things look.
1 komentar:
Why is this an exact copy of this post? http://www.noupe.com/ajax/create-a-simple-twitter-app.html
Posting Komentar